In this section, we will look at a class that implements a linked list of ListNode
objects. This class encapsulates the list operations that maintain the links as the list is modified. To keep the class simple, we will implement only a singly linked list, and the list class will supply direct access only to the first list element.
-
The SinglyLinkedList
class holds a reference, first
, to the first ListNode
(or null, if the list is completely empty). Access to the first node is provided by the getFirst
method. If the list is empty, a NoSuchElementException
is thrown (see Lesson A13, Exceptions).
public class SinglyLinkedList{
private ListNode first;
public SinglyLinkedList(){
first = null;
}
public Object getFirst(){
if (first == null){
throw new NoSuchElementException();
}else
return first.getValue();
}
}
-
Additional nodes are added to the head of the list with the addFirst
method. When a new link is added to the list, it becomes the head of the list, and the link that the old list had becomes the next link:
public class SinglyLinkedList{
...
public void addFirst(Object value) {
first = new ListNode(value, first);
}
...
}
-
The statement ListNode(value, first)
invokes the ListNode constructor. The line of code
first = new ListNode(value, first);
is broken down as follows.
-
The new
command allocates enough memory to store a ListNode
.
-
The new ListNode
will be assigned the values of value
and first
-
The address of this newly constructed ListNode
is assigned to first
.
-
It is important to understand the old and new values of first
:
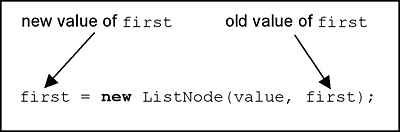
The very first time that addFirst()
is called, the instance variable, first, will be null. A new node is constructed with the values value and null
and now first
points to this new node. The constructor provides a new node between the variable first
and the node that first
formerly referenced.
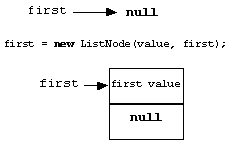 |
before the call of the constructor
call the constructor, first is passed as a null value
first is changed, references the newly constructed node
|
The second time that addFirst()
is called, first is already pointing to the first
node of the linked list. When the constructor is called, the new node is constructed and placed between first
and the node first
used to point to.
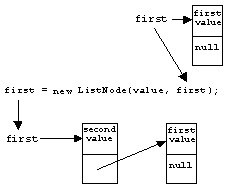
The value of first
passed to the ListNode
constructor is used to initialize the next
field of the new node.